This time I bring to all of you, the solution of a well-known algorithm and often used in technical interviews, it isn't hard to solve at all but it can put you in trouble if you don't practice solving algorithms. So let's get started.
Problem Statement
Given a number, I want to get all its divisors, for instance:
The function Divisors(10) must return: 1,2,4,10.
Building the Algorithm
What we are going to do is the following steps:
- Declare a list, this will be the response
- Iterate from 1 to the given number
- Check the remainder (% in Javascript) for each iteration
- If the remainder is zero then add it to the response list
- Return the list
Easy peasy huh? 😄 Let's code in JS:
function Divisores(limite){
var listaRespuesta = [];//response list declaration
for(let i = 1; i<= limite ; i++){
if(limite % i == 0)
{
listaRespuesta.push(i);//if the remainder is zero then add to the list
}
}
return listaRespuesta;
}
console.log(Divisores(10)); //making a test with 10 value
Testing
Here I will use Visual Studio Code with NodeJS for executing JavaScript in the console, let's see:
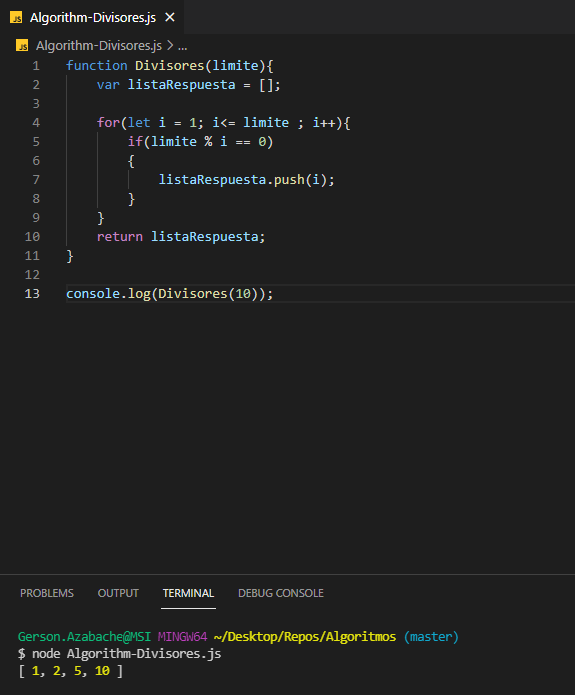
So now you know how you can solve this algorithm, practice a lot and get more confidence 😊
You can download the codebase in my Github account https://github.com/GeaSmart/algorithm-divisores
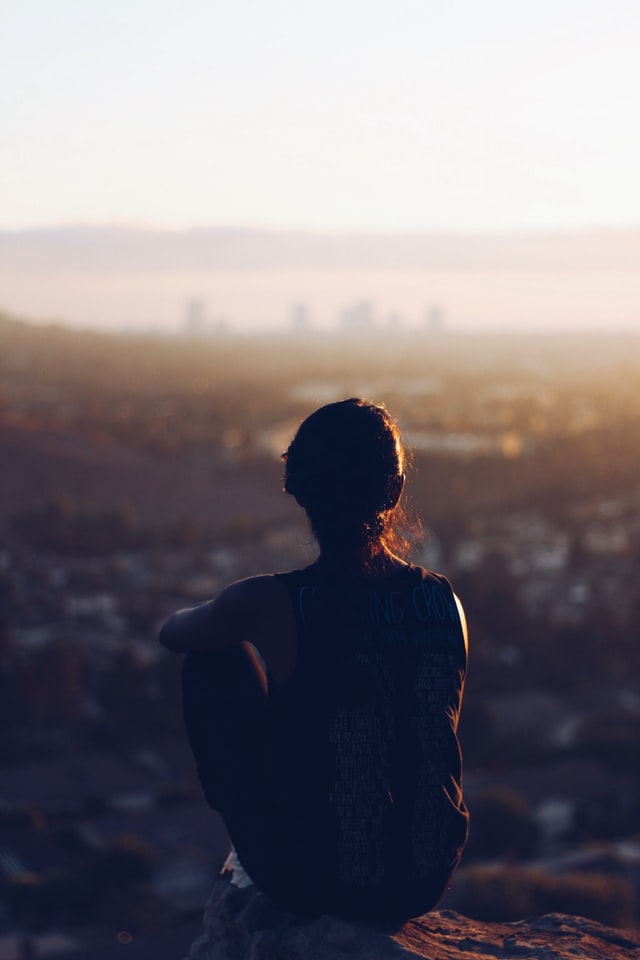
If you liked this post consider sharing it on your social media!