It's well known that the programming and technology field is rising high. If you want to follow this professional path or if your profession is related to tech and programming and you want to learn more about this or go deeper to improve your skills you may want to learn some definitions and general knowledge, this is the reason I write this post, I will try to condense the first steps you should take and the definitions you have to know and understand.
What is programming
This is the skill that allows you to communicate with a computer or a system and involves design, coding, testing, and deploy the application, this application can be desktop, mobile, or web. There are many other types but these three are the most important and known.
The systems communicate with humans through a programming language, there are several programming languages nowadays and they have their targets, for example, if you want to develop a desktop system, you can use Java or C#, if you prefer web apps you can choose PHP, Java, C#, Javascript among others. If your preferred field is machine learning your choice probably is going to be Python.
As you can see, mostly a project involves the use of many languages not only one.
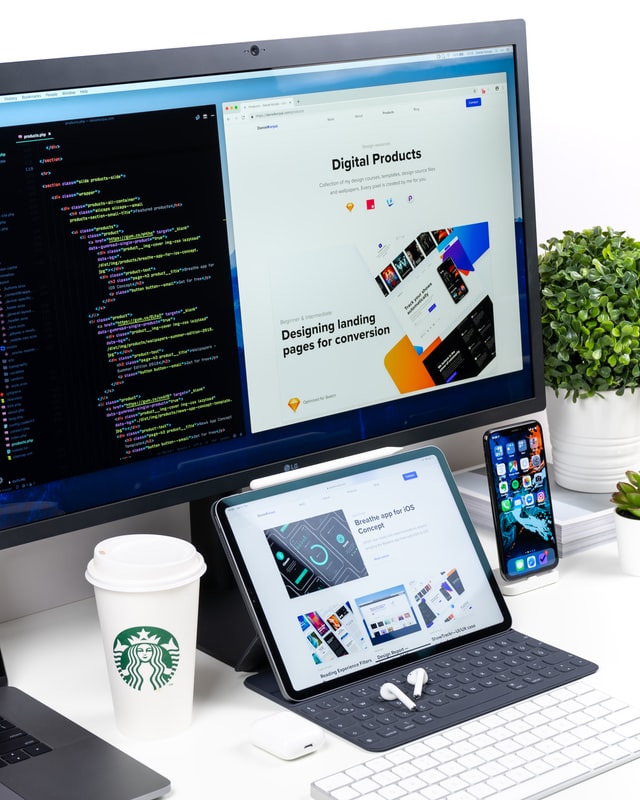
Web Development
Typically this is the programming specialization that most people use to start their programming career, this is because you can progress quicker than desktop or mobile, and the learning curve is not so high, you can learn the very basics and then continue acquiring the knowledge that allows you to make more complex applications.
Hey buddy, let's discuss the very basics in this field 😉
The very basics
In Web development typically the most simple project you can choose to start is a static web page, for example, a corporate information web page for a business in your city. Let's say a pharmacy.
The first step for a pharmacy to start its presence on the Internet is to create an informative web page. You as a new web developer can help out with this.
After some time, the pharmacy may want to sell its products over its web page, this is one more complex step, and this requires not an informative web page, but a web application instead because this uses a database, there is business logic involved, some calculation in the backend is needed and a back-office dashboard, shopping cart and other features that, as you can see, add a level of complexity, this is why is called Web Application instead of a page.
Concepts
We have said many concepts that a developer uses in its day-to-day life and you may want to learn, for example:
- Front-end: This is the visible part of a web application includes the UI and UX (user interface and experience)
- Back-end: This is the computing part of the system, and is not visible directly for the user, this calculation is done in the server.
- Business Logic: The logic and the way of calculation for a process in every business, for example how the discount function in a sales system, or the way the payroll is calculated in a payroll system.
- Database: A repostory where data is stored, tipically resides in the Server.
- Web page: A web that shows information to a user about a company or service
- Web application: A web system that interacts with the user and stores and retrieves data, contains sessions and business logic.
Understanding a simple web page
Every web page is basically made of HTML, CSS, and JAVASCRIPT, but for starting out you only need knowledge in HTML and CSS.
HTML File
This file sets the layout of the page.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=, initial-scale=1.0">
<link rel="stylesheet" href="styles.css">
<title>Simple Web Page</title>
</head>
<body>
<header>
<div class="container">
<h1>My Web Page</h1>
<nav>
<ul>
<li><a href="">Home</a></li>
<li><a href="">Gallery</a></li>
<li><a href="">About</a></li>
</ul>
</nav>
</div>
</header>
<main class="container content">
<article>
<section>
<h2>My section</h2>
<p>Lorem ipsum dolor sit amet consectetur adipisicing elit. Exercitationem, eveniet odit? Nobis impedit autem possimus nesciunt nam quo laboriosam doloremque optio eveniet. Iste ipsam nisi praesentium quasi sed! Alias, iure.</p>
<img src="" alt="">
</section>
<section>
<h2>My section</h2>
<p>
Lorem ipsum dolor sit amet consectetur adipisicing elit. Exercitationem, eveniet odit? Nobis impedit autem possimus nesciunt nam quo laboriosam doloremque optio eveniet. Iste ipsam nisi praesentium quasi sed! Alias, iure.
Lorem ipsum dolor sit amet consectetur adipisicing elit. Exercitationem, eveniet odit? Nobis impedit autem possimus nesciunt nam quo laboriosam doloremque optio eveniet. Iste ipsam nisi praesentium quasi sed! Alias, iure.
Lorem ipsum dolor sit amet consectetur adipisicing elit. Exercitationem, eveniet odit? Nobis impedit autem possimus nesciunt nam quo laboriosam doloremque optio eveniet. Iste ipsam nisi praesentium quasi sed! Alias, iure.
</p>
<img src="" alt="">
</section>
<section>
<h2>My section</h2>
<p>Lorem ipsum dolor sit amet consectetur adipisicing elit. Exercitationem, eveniet odit? Nobis impedit autem possimus nesciunt nam quo laboriosam doloremque optio eveniet. Iste ipsam nisi praesentium quasi sed! Alias, iure.</p>
<img src="" alt="">
</section>
</article>
<aside>
<ul>
<li><a href="">Link 1</a></li>
<li><a href="">Link 2</a></li>
<li><a href="">Link 3</a></li>
</ul>
</aside>
</main>
<footer>
<p>Copyright - 2021</p>
</footer>
</body>
</html>
Styles in CSS
This file sets the style of the page
*{
margin:0;
padding: 0;
box-sizing: border-box;
}
.container{
width: 75%;
margin: 0 auto;
}
header{
background-color: black;
height: 110px;
}
header h1{
color: white;
margin-bottom: 15px;
padding-top: 10px;
}
nav ul{
list-style: none;
}
ul{
list-style: none;
}
nav ul li{
display: inline-block;
width: 120px;
background-color: yellow;
height: 30px;
text-align: center;
line-height: 30px;
}
nav ul li a{
color:black;
text-decoration: none;
}
body{
min-height: 100%;
font-family: Verdana, Geneva, Tahoma, sans-serif;
}
.content{
background-color: aquamarine;
margin: 0 auto;
min-height: 750px;
}
article{
width: 70%;
float: left;
padding: 20px;
}
aside{
width: 30%;
float: right;
background-color: blanchedalmond;
height: 750px;
padding: 15px;
}
footer{
background-color: black;
height: 80px;
color:white;
text-align: center;
line-height: 80px;
font-weight: bold;
}
Both documents together in Visual Studio Code look like this
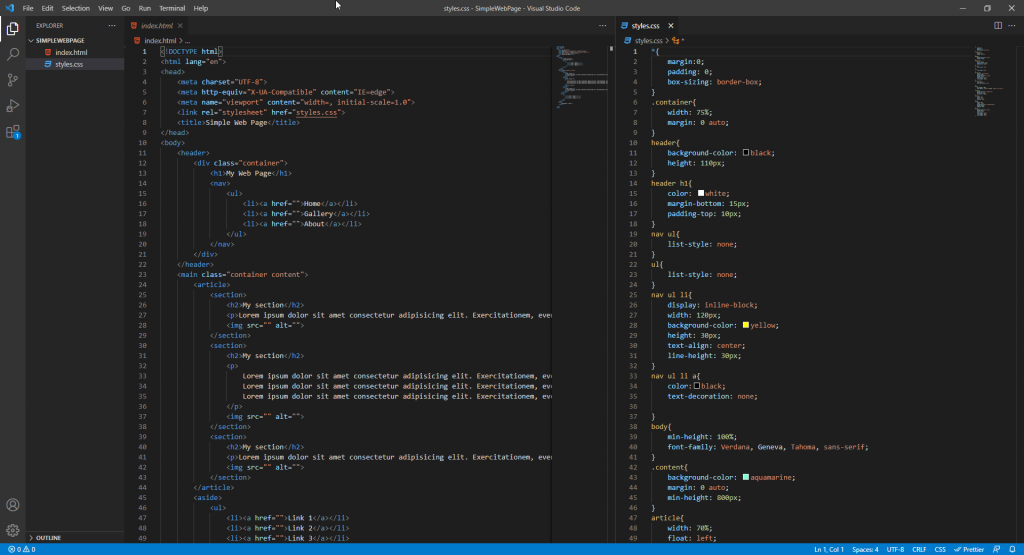
The result if we open the HTML document is this:
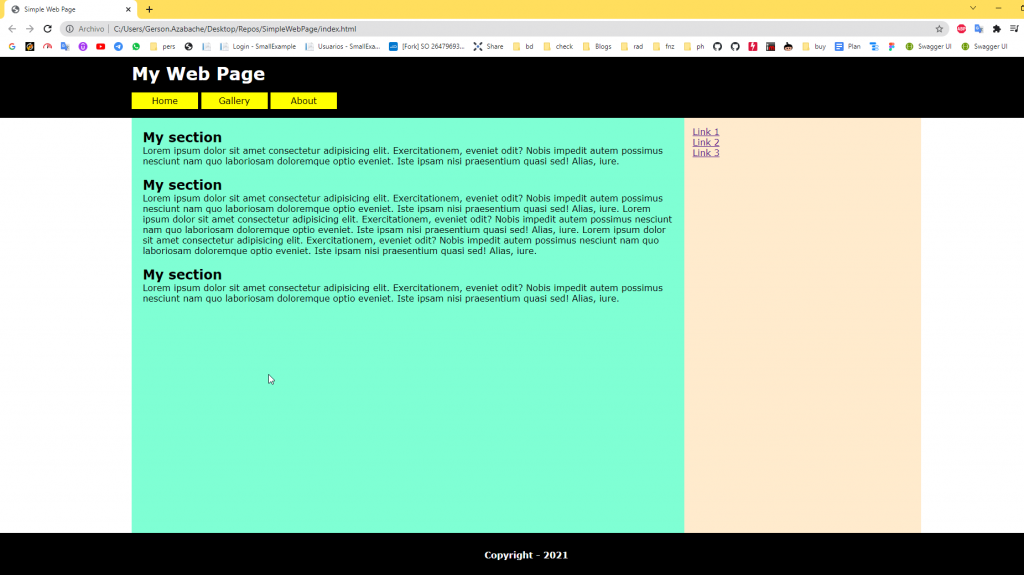
This is a basic web page, as you can see only HTML and CSS were needed.
This is the first step in web development.
So you have to learn HTML, CSS and with several web pages done, learn Javascript, then you can consider yourself a frontend developer.
Then you can learn a front-end framework such as Angular, React, or Vue to be a more complete developer.
Then you can add backend technology to your skills, but that's for another post.
This is a wonderful career path, if you read all this post and made your first web page, this is for you:
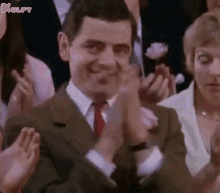
Hey dude, If you liked this post, consider sharing this on your social media 🔥😉
Thank you for this valuable material!!
Thanks for your support, keep pushing forward Angelina! 💪
hello there and thank you for your information – I’ve certainly picked up
something new from right here.